ID Scanner API
AI-Powered ID Scanning
Transform ID Verification
with AI Intelligence
Streamline your ID verification process with our powerful AI technology. Built for businesses that need reliable, automated document processing with enterprise-grade accuracy.
99.9% Accuracy
Industry-leading precision in data extraction
Fast Processing
Efficient ID scanning within seconds
Enterprise Security
Bank-grade encryption and compliance
Image Guidelines
For best results, please follow these guidelines when submitting ID images:
Good Examples
Follow these guidelines
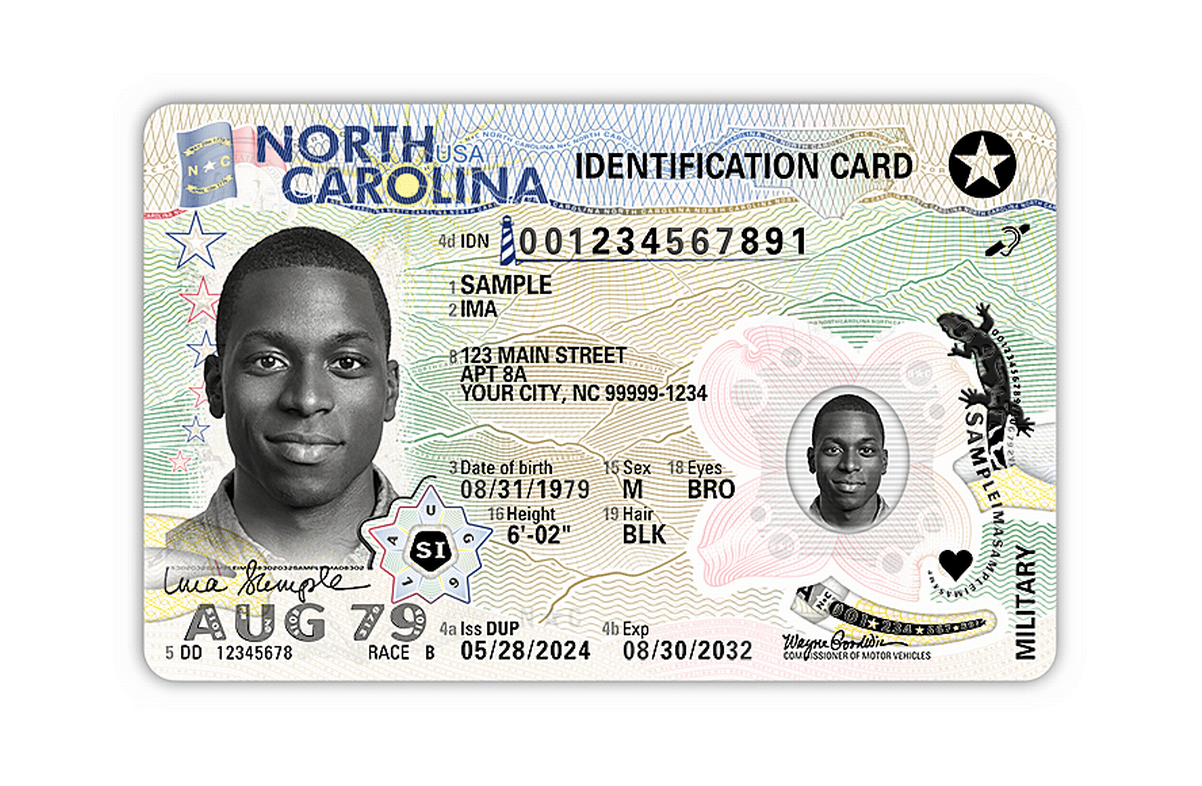
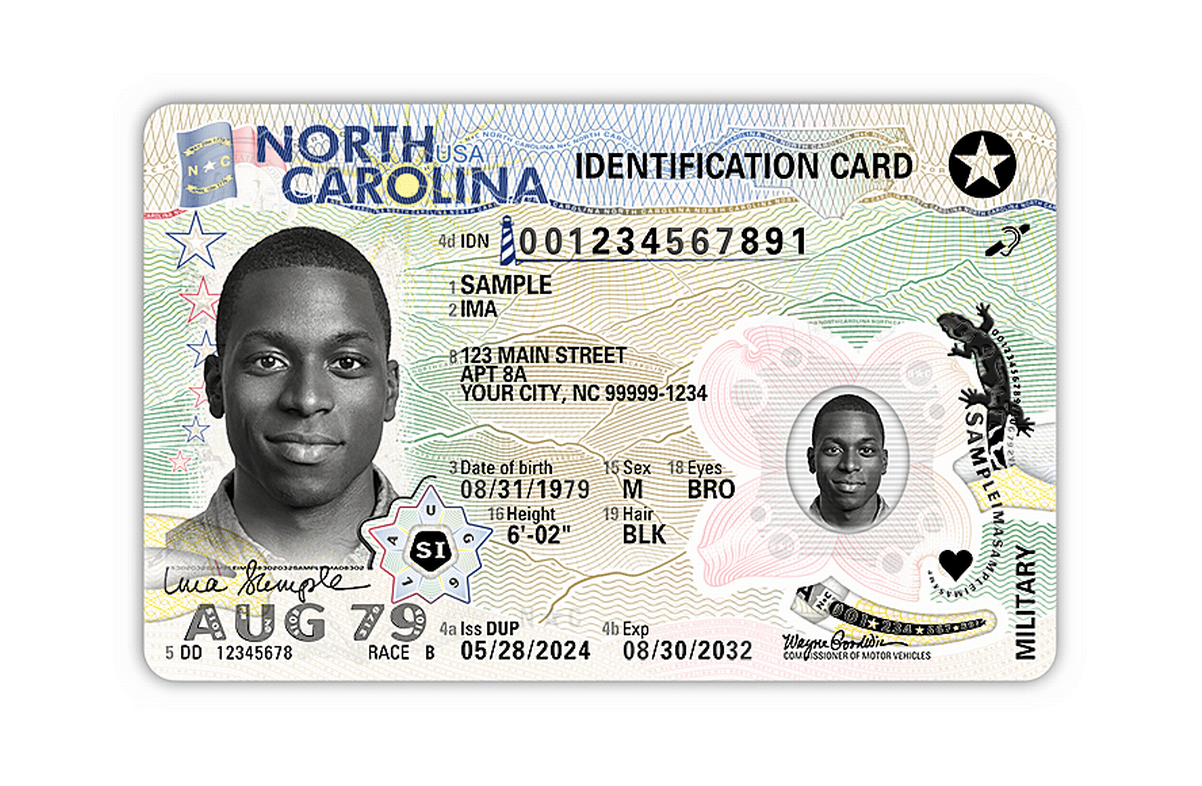
Common Mistakes
What to avoid
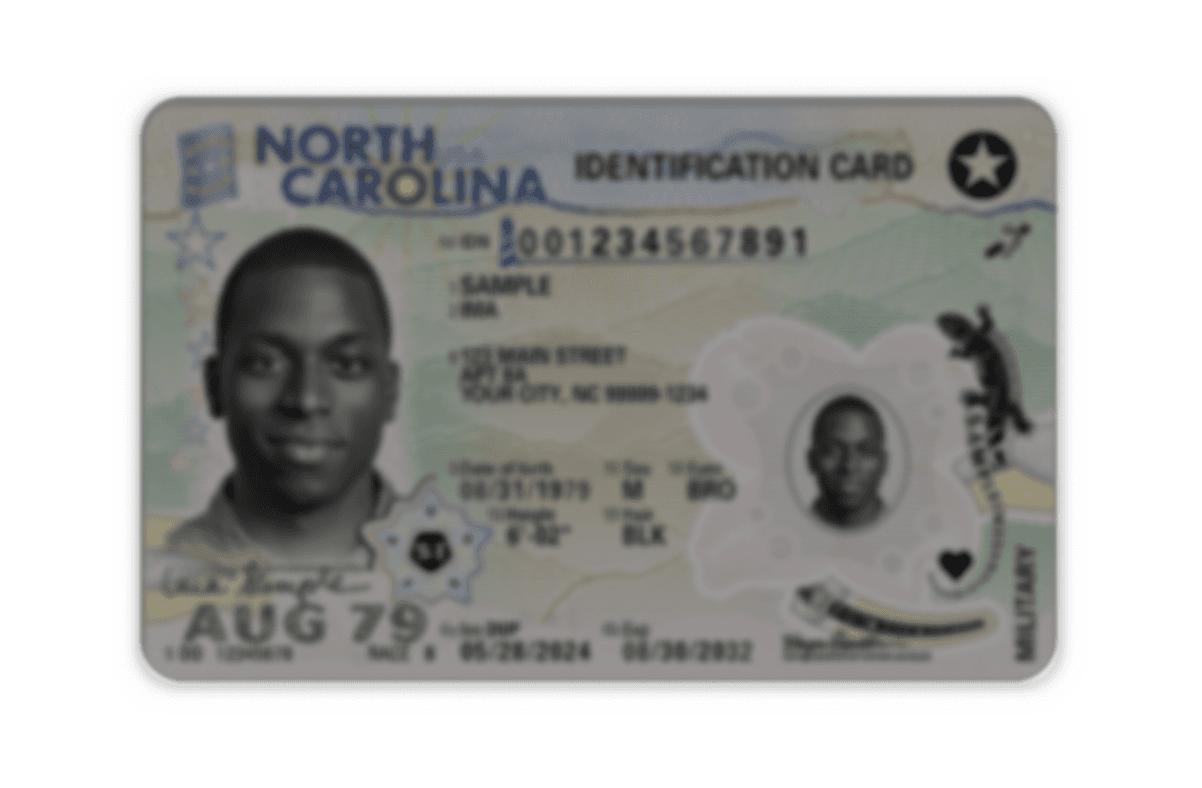
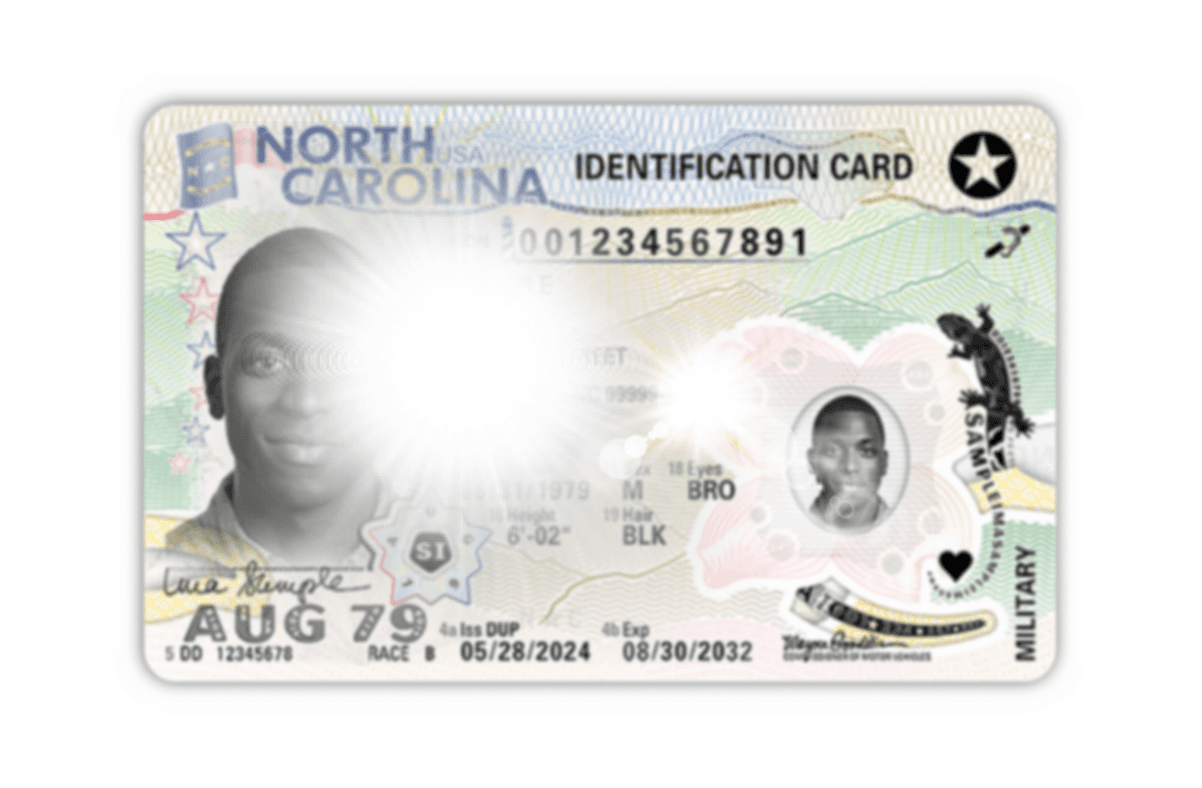
Pricing
Choose the perfect plan for your business needs. Scale up or down as your requirements change.
Starter
- 100 ID scans
- Basic support
- API access
Professional
- 500 ID scans
- Priority support
- API access
- Webhook notifications
Enterprise
- 2000 ID scans
- API access
- Webhook notifications
- Custom integration help
Enterprise Custom Plan
Tailored solutions for large-scale operations and specific business needs
Everything in Enterprise, plus:
Dashboard Experience
Take control of your API usage with our powerful dashboard interface.
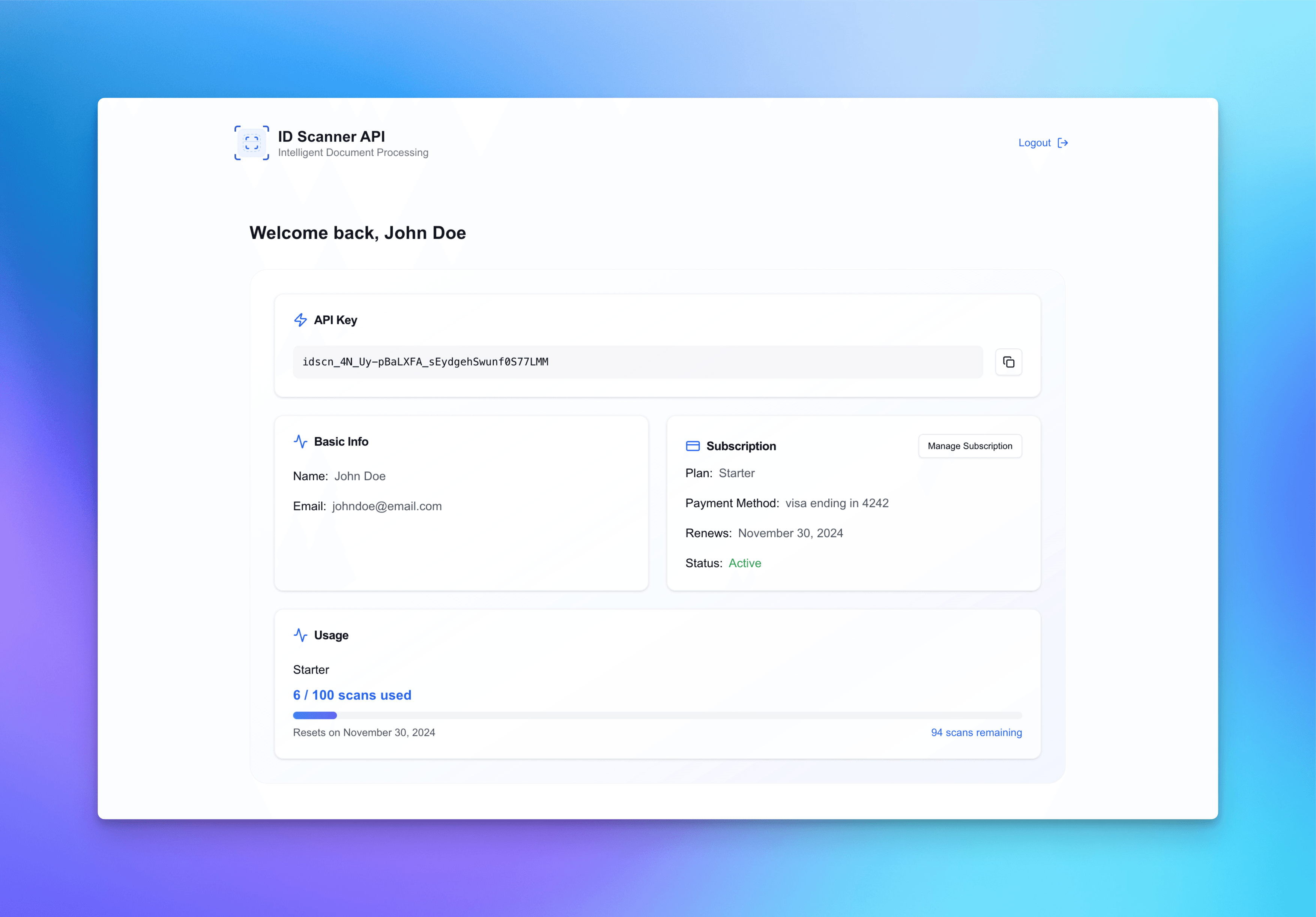
Powerful Dashboard Interface
Get instant access to your API keys, usage metrics, and subscription management through our intuitive dashboard.
API Key Management
Securely access and manage your API keys
Usage Monitoring
Track your API usage with real-time metrics
Subscription Control
Easily manage your subscription and billing
API Documentation
Authentication
Include your API key in the request headers:
x-api-key: your_api_key_here
Endpoint
Send your requests to this endpoint:
POST /api/scan
Request
Two ways to send your request:
1. File Upload
Send a multipart/form-data request with:
- image: File (JPEG or PNG, max 20MB)
2. Image URL
Send a JSON request with:
{
"imageUrl": "https://example.com/image.jpg"
}
Code Examples
Curl
curl -X POST \
-H "Authorization: Bearer your_api_key_here" \
-F "image=@/path/to/your/image.jpg" \
https://api.yourdomain.com/api/scan
curl -X POST \
-H "Authorization: Bearer your_api_key_here" \
-H "Content-Type: application/json" \
-d '{"imageUrl": "https://example.com/image.jpg"}' \
https://api.yourdomain.com/api/scan
Python
import requests
url = "https://api.yourdomain.com/api/scan"
headers = {"Authorization": "Bearer your_api_key_here"}
files = {"image": open("image.jpg", "rb")}
response = requests.post(url, headers=headers, files=files)
print(response.json())
import requests
url = "https://api.yourdomain.com/api/scan"
headers = {
"Authorization": "Bearer your_api_key_here",
"Content-Type": "application/json"
}
data = {"imageUrl": "https://example.com/image.jpg"}
response = requests.post(url, headers=headers, json=data)
print(response.json())
Javascript
const axios = require('axios');
const FormData = require('form-data');
const fs = require('fs');
const form = new FormData();
form.append('image', fs.createReadStream('image.jpg'));
axios.post('https://api.yourdomain.com/api/scan', form, {
headers: {
...form.getHeaders(),
'Authorization': 'Bearer your_api_key_here'
}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
const axios = require('axios');
axios.post('https://api.yourdomain.com/api/scan', {
imageUrl: 'https://example.com/image.jpg'
}, {
headers: {
'Authorization': 'Bearer your_api_key_here',
'Content-Type': 'application/json'
}
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
Response Examples
Success Response (200)
{
"success": true,
"status": 200,
"data": {
"document_type": "Driver License",
"DL_number": "D1234567",
"first_name": "John",
"last_name": "Doe",
"state": "California",
"address": {
"street": "123 Main St",
"city": "Los Angeles",
"state": "CA",
"zip_code": "90001"
},
"USA": true,
"issue_date": "2020-01-01",
"expiration_date": "2025-01-01",
"date_of_birth": "1990-05-15",
"restrictions": "None",
"class": "C",
"endorsements": "None",
"sex": "M",
"height": "5'10\"",
"weight": "180 lbs",
"hair_color": "Brown",
"eye_color": "Blue",
"DD_number": "123456789"
}
}
Fields that cannot be found in the ID or don't have a matching value will be returned as null
in the response.
Unreadable Image (422)
{
"success": false,
"status": 422,
"error": "Image is not clear enough",
"details": "Please provide a clearer image of the ID"
}
Invalid Document (400)
{
"success": false,
"status": 400,
"error": "Not an ID document",
"details": "The image provided does not appear to be an ID document"
}
Suspicious Document (403)
{
"success": false,
"status": 403,
"error": "Suspicious document",
"details": "The document appears to be modified or invalid"
}
Unauthorized (401)
{
"success": false,
"status": 401,
"error": "Missing or invalid API key"
}
Usage Limit Exceeded (429)
{
"success": false,
"status": 429,
"error": "Usage limit exceeded",
"details": "Plan limit: 100, Current usage: 100"
}
Frequently Asked Questions
Find answers to common questions about our ID Scanner API.